Note
Go to the end to download the full example code.
Shape Interpolation#
Given two shapes as triangulated 3D models, how can we smoothly interpolate another model between them using signed distance fields
import numpy as np
import pyvista as pv
import mirage as mr
Animating the entire interpolation
obj1 = mr.SpaceObject('icosahedron.obj').clean()
obj2 = mr.SpaceObject('duck.obj').clean()
pl = pv.Plotter()
pl.open_gif('shape_interpolation.gif')
for frac1 in np.concatenate((np.linspace(0, 1, 20), np.linspace(1, 0, 20))):
weights = np.array([1 - frac1, frac1]).astype(float)
mr.tic()
obj_merged = mr.merge_shapes([obj1, obj2], weights)
mr.toc()
pl.add_mesh(obj_merged._mesh, color='lightblue', name='mesh', smooth_shading=True)
pl.add_text(
f'{weights[0]*100:3.0f}% Icosahedron \n{weights[1]*100:3.0f}% Duck',
font='courier',
name='label',
)
pl.write_frame()
pl.close()
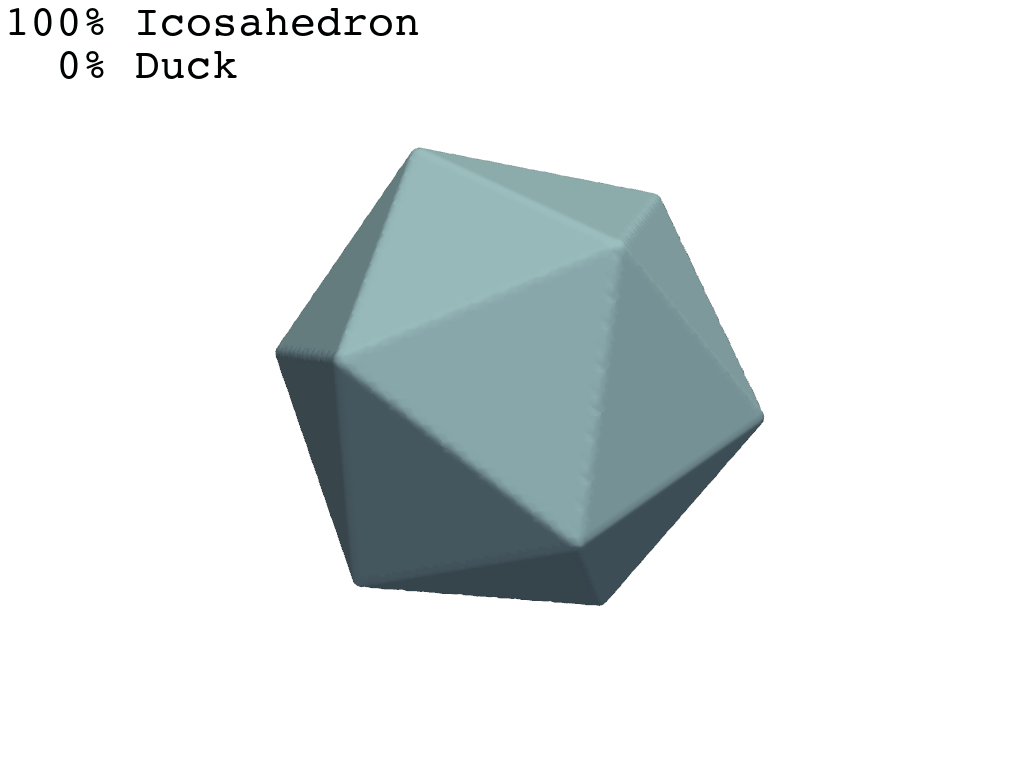
Elapsed time: 5.23e-01 seconds
Elapsed time: 4.90e-01 seconds
Elapsed time: 4.68e-01 seconds
Elapsed time: 4.77e-01 seconds
Elapsed time: 4.92e-01 seconds
Elapsed time: 4.73e-01 seconds
Elapsed time: 4.75e-01 seconds
Elapsed time: 4.58e-01 seconds
Elapsed time: 4.81e-01 seconds
Elapsed time: 5.24e-01 seconds
Elapsed time: 4.97e-01 seconds
Elapsed time: 4.82e-01 seconds
Elapsed time: 5.20e-01 seconds
Elapsed time: 4.72e-01 seconds
Elapsed time: 4.86e-01 seconds
Elapsed time: 4.46e-01 seconds
Elapsed time: 4.56e-01 seconds
Elapsed time: 4.55e-01 seconds
Elapsed time: 4.54e-01 seconds
Elapsed time: 4.59e-01 seconds
Elapsed time: 4.64e-01 seconds
Elapsed time: 4.44e-01 seconds
Elapsed time: 4.69e-01 seconds
Elapsed time: 4.60e-01 seconds
Elapsed time: 4.65e-01 seconds
Elapsed time: 4.50e-01 seconds
Elapsed time: 4.53e-01 seconds
Elapsed time: 4.42e-01 seconds
Elapsed time: 4.60e-01 seconds
Elapsed time: 4.53e-01 seconds
Elapsed time: 5.08e-01 seconds
Elapsed time: 5.01e-01 seconds
Elapsed time: 4.92e-01 seconds
Elapsed time: 4.92e-01 seconds
Elapsed time: 5.22e-01 seconds
Elapsed time: 4.82e-01 seconds
Elapsed time: 4.96e-01 seconds
Elapsed time: 4.69e-01 seconds
Elapsed time: 4.70e-01 seconds
Elapsed time: 4.73e-01 seconds
Individual interpolation steps in a grid
pl = pv.Plotter(shape=(2, 2))
for i, weight1 in enumerate(np.linspace(0, 1, 4)):
weights = np.array([1 - weight1, weight1]).astype(float)
obj_merged = mr.merge_shapes(
[
mr.SpaceObject('icosahedron.obj').clean(),
mr.SpaceObject('torus.obj').clean(),
],
weights,
)
pl.subplot(i // 2, i % 2)
pl.add_mesh(obj_merged._mesh, color='lightblue', name='mesh', smooth_shading=True)
pl.add_text(
f'{weights[0]*100:3.0f}% Icosahedron \n{weights[1]*100:3.0f}% Torus',
font='courier',
name='label',
)
pl.show()
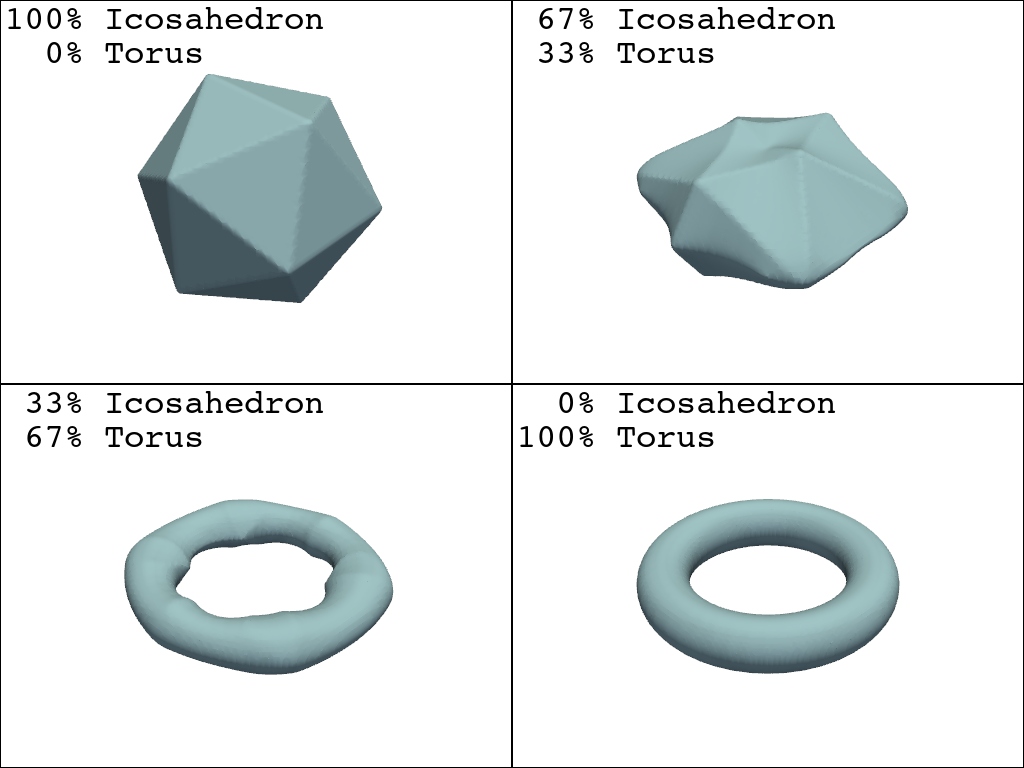
Model file torus.obj not found in current model folder ('/Users/liamrobinson/Documents/maintained-research/mirage/mirage/resources/models'), checking model repository...
Attempting to download torus.obj and its associated material file from the model repository...
Requesting: https://raw.githubusercontent.com/ljrobins/mirage-models/main//Non-Convex/torus.obj
Requesting: https://raw.githubusercontent.com/ljrobins/mirage-models/main//Non-Convex/torus.mtl
Model files were downloaded successfully!
Total running time of the script: (0 minutes 26.085 seconds)